Ads
This package simplifies showing ads from Max SDK.
It automatically handles:
- loading the ads
- sending required Lion Analytics Ad events
⚠️ |
Do not call Lion Analytics’ Ad events if you use Lion Ads, or they will be sent twice. |
Setup
- Go to LionStudios→Settings Manager in the Unity menu
- Switch to the Ads tab
- Turn ON the “Enable Lion Ads” checkbox
- Fill up the required ad unit IDs
- Leave the other settings to their default values unless requested
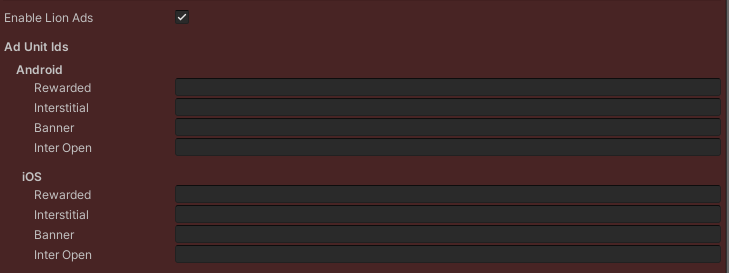
👉 |
Google Ads Manager requires its own set of IDs; enter those IDs in the MAX Integration Manager if Google Ads Manager is installed as an adapter. ![]() |
Usage
Lion Ads provides just a few static functions to show and handle ads.
-
LionAds.IsRewardedReady
- returns:
boolean
Call this to decide whether to propose rewarded buttons or to disable them
- returns:
-
LionAds.TryShowRewarded(string placement, Action onRewarded, Action onClosed = null, Reward reward = null, Dictionary<string, object> additionalData)
- returns:
boolean
: false if no ad was ready - parameters:
placement
: a string describing this ad placement (ie: “SkinShop”, “SkipLevel”…)onRewarded
: a callback called when the player has watched through the ad. This should contain the code that gives the actual reward to the player.onClosed
(optional): a callback called when the ad was closed (completed or canceled). This can be used to resume normal flow after the ad.reward
(optional): this object will be passed in the automated call toLionAnalytics.RewardVideoCollect
. (cf Ad Events)additionalData
(optional): this parameter will be passed to all ad events related to this ad (that is all RewardVideoX events except _Load and _LoadFail).
Call this to show a rewarded video if available.
- returns:
-
LionAds.TryShowInterstitial(string placement, Action onClosed = null, Dictionary<string, object> additionalData)
- returns:
boolean
: false if no ad was ready - parameters:
placement
: a string describing this ad placement (ie: “LevelEnd”…)onClosed
(optional): a callback called when the ad was closed (completed or canceled or not ready). This can be used to resume normal flow after the ad.additionalData
(optional): this parameter will be passed to all ad events related to this ad (that is all InterstitialX events except _Load and _LoadFail).
Call this to show an interstitial video if available.
- returns:
-
LionAds.TryShowInterOpen(string placement, Action onClosed = null, Dictionary<string, object> additionalData = null)
- returns:
boolean
: false if no ad was ready - parameters:
placement
: a string describing this ad placement (ie: “AppOpened”…)onClosed
(optional): a callback called when the ad was closed (completed or canceled or not ready). This can be used to resume normal flow after the ad.additionalData
(optional): this parameter will be passed to all ad events related to this ad (that is all InterOpenX events except _Load and _LoadFail).
- returns:
-
LionAds.ShowBanner()
- returns:
boolean
: false if no ad was ready. Even if false is returned, it will still load and show the banner as soon as ready.
Call this to show a banner ad as soon as available.
The banner will be positioned according to the “Banner Position” setting.
- returns:
-
LionAds.HideBanner()
- returns:
void
Call this to hide the banner ad.
- returns:
-
LionAds.OnRewardedStatusChanged
- type:
Action<bool>
Subscribe to this event to update RV buttons status when a Rewarded becomes ready. This is to avoid having to call
IsRewardedReady
constantly. - type: