Options Menu
An options menu prefab with options that games typically need. These features include the ability to control audio and haptic, GDPR via a privacy button, a restore purchases button, a feedback button, social links and user information.
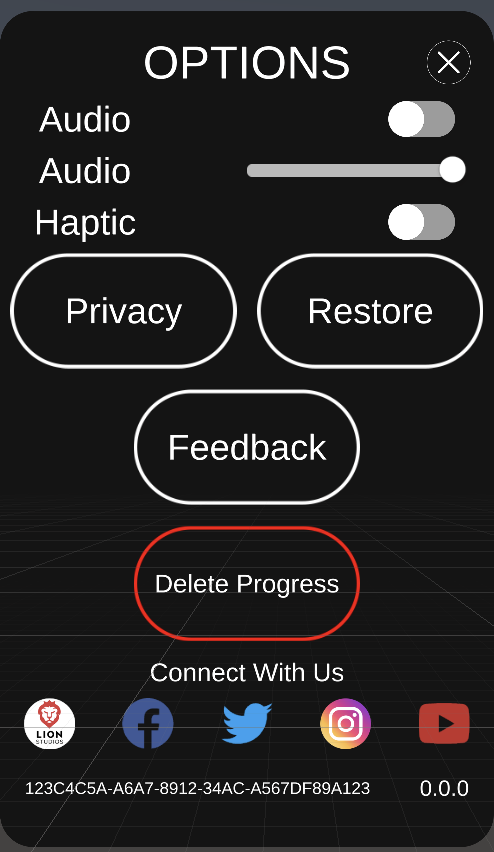
Installation
- Complete the steps in Getting Started
⚠️ |
Make sure the “Hide Package” button is disabled to see the relevant package folders. ![]() |
Integration
-
Go to
Packages/Lion - Options Menu/Prefabs
-
Drag and drop
OpenMenuButton.prefab
in your UI canvas*OpenMenuButton
is a simple UI button that opensLionOptionsCanvas
-
Position the button appropriately in your UI
-
Implement the RestorePurchase callback
LionOptionsMenu.RestorePurchase += () => { //Handle restore purchase here };
Optional steps:
- Go to
Packages/Lion - Options Menu/Prefabs/Menu/Resources
- Drag and drop
LionOptionsCanvas.prefab
in your scene*LionOptionsCanvas
is a self-sufficient canvas that contains the usual game options.
- Customize the UI of the button and menu to your needs
⚠️ |
![]() |
💡 |
If the canvas prefab is not imported in the scene, the default prefab will be automatically instantiated at runtime when the OpenMenuButton is clicked.
|
Settings
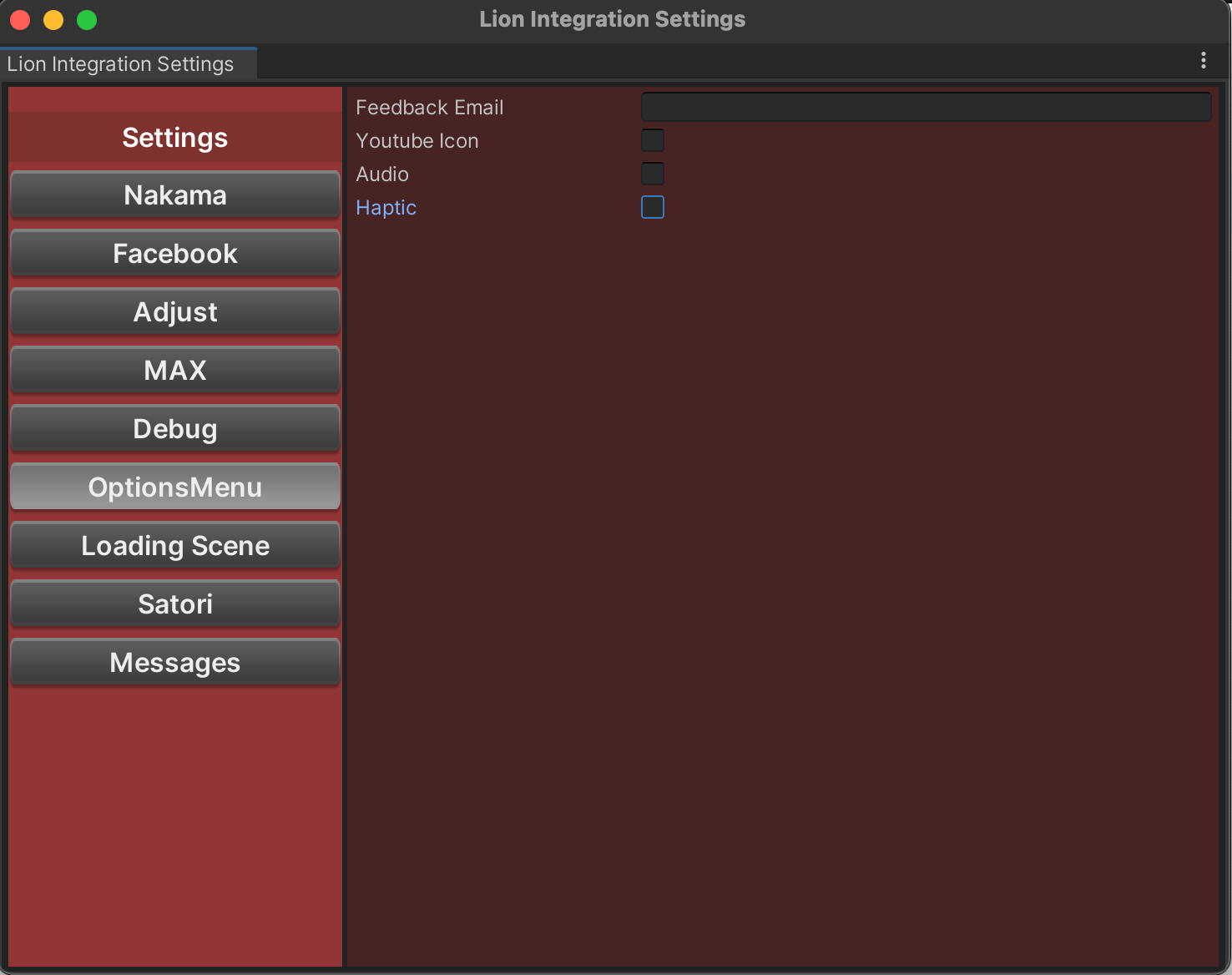
- Settings included in
LionStudios -> Settings Manager -> Options Menu
:-
Feedback Email:
- ⚠️ This setting will only be used if Helpshift is not installed Helpshift - Customer Support
- This email will be used as the receiver address for the “Feedback button”, which opens the user’s email app and allows them to directly provide feedback about the app. It should be set to the developer’s email. If left empty, hello@lionstudios.cc will be used.
-
Social Buttons:
- Enable this to display the “Connect with us” section, which provides links to our social networks. This should be disabled for games that are not officially on Lion Studios account yet.
-
Youtube Icon:
- Enable this to display the Youtube social button. This is disabled by default because Google sometimes rejects games with youtube links if it thinks they are appealing to kids.
-
Audio:
-
Enable this to display the Audio Button in the menu.
-
Audio Option Type
- Toggle: the audio option will be a On/Off toggle
- Slider: the audio option will be a slider, allowing volume control
-
-
Haptic:
- Enable this to display the Haptic Button in the menu.
-
Advanced Setup
You can also import option menu elements independently, to integrate them inside an already existing Options UI.
Following are the prefabs/elements that can be used independently. You can use the prefab as it is or create a prefab variant.
To create prefab variants, please follow this guide: Change the reference in the Leaderboard Panel prefab variant, from original Leaderboard Entry prefab to the new Leaderboard Entry prefab variant that you have just created
All menu element prefabs:
These prefabs can be found under Packages/Lion - Options Menu/Prefabs/MenuElements/
- SoundSlider.prefab
- This slider will control the game’s AudioListener volume
- The value will be saved to the device and reloaded whenever the menu is reopened on a future session.
- To access this value use:
SoundSlider.status
- SoundButton.prefab
- This button will control the game’s AudioListener’s toggle
- The value will be saved to the device and reloaded whenever the menu is reopened on a future session
- To access this value, use:
SoundButton.status
- To detect value changes, use the callback
LionOptionsMenu.OnAudioToggleChanged
- HapticButton.prefab
- This button will control the game’s Haptic toggle
- Currently , All Lion games use Feel as their Vibration and haptic control asset, so the code to control those properties from FEEL have been included to the button functionality.
- The value will be saved to the device and reloaded whenever the menu is reopened on a future session.
- To detect value changes, use the callback
LionOptionsMenu.OnHapticToggleChanged
- PrivacyButton.prefab
- This button will only appear in GDPR compliant countries.
- When clicked, it will showcase the Max Consent flow privacy popup, and invoke the
LionOptionsMenu.PrivacyButtonClicked
callback.
- RestoreButton.prefab
- This button will invoke an event callback (RestorePurchase) that developers can subscribe to and perform their restore purchase functionality (See Public Events ).
- This button will only appear on iOS devices.
- To detect if the button is clicked, use the callback
LionOptionsMenu.RestorePurchase
- FeedbackButton.prefab
- If helpshift is installed (Helpshift - Customer Support)
- Helpshift support window will be opened
- If helpshift is not installed
- The player mail app will be opened with a prefilled email that will be directed to the developers email, and contains useful info (version number, device ADID, and IDFV).
- The dev’s email is set via the Settings Manager. More detail ahead in “ Configuring the Options Menu Settings” part.
- To detect if the button is clicked, use the callback
LionOptionsMenu.OnFeedbackButtonClicked
- If helpshift is installed (Helpshift - Customer Support)
- AppInfo.prefab
- The bottom of the options panel includes information about the device and game. The first value is the ADID of the user. The text on the right includes the app version number.
- The ADID and version number have “tap to copy” functionality. Click on the numbers to copy them to your clipboard.
- LionSocials.prefab
- This container contains all the social links to the various Lion Studios Social platforms.
- Devs can use LionSocials prefab as a whole or they can also use social buttons prefab individually. Following are the buttons if prefabs are used individually,
- FacebookButton.prefab
- A simple image button that will open Lion Studios Facebook Page.
- InstagramButton.prefab
- A simple image button that will open Lion Studios instagram page.
- TwitterButton.prefab
- A simple image button that will open Lion Studios twitter page.
- WebButton.prefab
- A simple image button that will open Lion Studios web page.
- YoutubeButton.prefab
- A simple image button that will open Lion Studios youtube page
- FacebookButton.prefab
Delete Progress
The Delete Progress button supports user privacy, and gives the user the ability to start the game over.
When Delete Progress is pressed, it will show a confirmation popup:
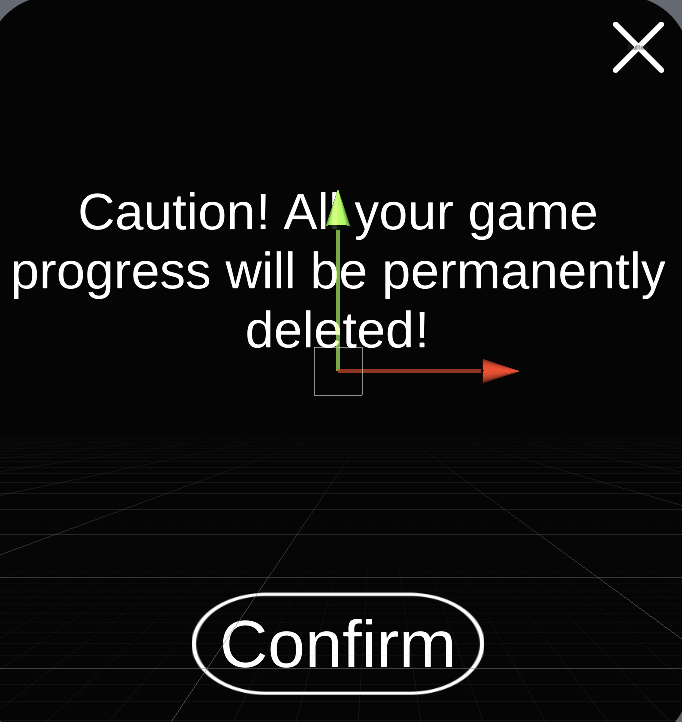
When the Confirm button is pressed:
- All data saved locally (PlayerPrefs) will be deleted
- All user data on the Nakama server will be deleted (if applicable)
- If a custom callback is subscribed by the game code, it will be executed
- On android, the app will restart. On iOS, the app will close
Custom callback
You can subscribe a custom callback to LionCore.OnDeleteProgressConfirmed
and it will be executed when confirm delete progress button is clicked.
Example:
private void Awake()
{
LionCore.OnDeleteProgressConfirmed += OnDeleteProgress;
}
private async Task OnDeleteProgress()
{
// Game specific delete progress code here
}
Code Features
Make sure to add the “using namespace LionStudios.Suite.OptionsMenu;”
Public Methods
- LionOptionsMenu.Instance.Show()
- Use this method to open the options menu popup by code
- LionOptionsMenu.Instance.Close()
- Use this method to close the options menu popup by code
Public Events
-
OnOptionsOpened
- This event will be invoked whenever the options panel is opened
-
OnOptionsClosed
- This event will be invoked whenever the options panel is closed
-
OnFeedbackButtonClicked
- This event will be invoked whenever the feedback button is clicked
-
OnPrivacyButtonClicked
- This event will be invoked whenever the privacy button is clicked
-
OnAudioToggleChanged
- This event will be invoked whenever audio button is clicked
-
OnHapticToggleChange
- This event will be invoked whenever haptic button is clicked
-
OnRestorePurchase
- This event will be invoked whenever a player clicks on the “Restore” button.
- Developers are expected to subscribe to this method and handle purchase restoration on their own
LionOptionsMenu.OnOptionsOpened += () => { Debug.Log("Lion Options menu is OPENED"); }; LionOptionsMenu.OnOptionsClosed += () => { Debug.Log("Lion Options menu is CLOSED"); }; LionOptionsMenu.OnFeedbackButtonClicked += () => { Debug.Log("Feedback button was clicked"); }; LionOptionsMenu.PrivacyButtonClicked += () => { Debug.Log("Privacy button clicked, do something..."); }; LionOptionsMenu.OnAudioToggleChanged += (audioStatus) => { Debug.Log("Audio toggle status changed, do something..."+audioStatus); }; LionOptionsMenu.OnHapticToggleChanged += (hapticStatus) => { Debug.Log("Haptic toggle status changed, do something..."+hapticStatus); }; LionOptionsMenu.RestorePurchase += () => { Debug.Log("Handle restore purchase here"); };